A move in Rust is one of those concepts that’s unfamiliar to many programmers coming from C#, Javascript, and similar garbage-collected languages, and different from what you’re used to for C and C++ programmers. The definition of move in Rust is closely related to its ownership system.
Moving means transferring ownership. In Rust, a move is the default way of passing values around and it happens every time you change ownership over an object. If the object you move only consists of copy types (types that implement the Copy trait), this is as simple as copying the data over to a new location on the stack.
For non-copy types, a move will copy all copy types that it contains over just like in the first example, but now, it will also copy pointers to resources such as heap allocations. The moved-from object is left inaccessible to us (for example, if you try to use the moved-from object, the compilation will fail and let you know that the object has moved), so there is only one owner over the allocation at any point in time.
In contrast to cloning, it does not recreate any resources and make a clone of them.
One more important thing is that the compiler makes sure that drop is never called on the moved-from object so that the only thing that can free the resources is the new object that took ownership over everything.
Figure 9.2 provides a simplified visual overview of the difference between move, clone, and copy (we’ve excluded any internal padding of the struct in this visualization). Here, we assume that we have a struct that holds two fields – a copy type, a, which is an i64 type, and a non-copy type, b, which is a Vec<u8> type:
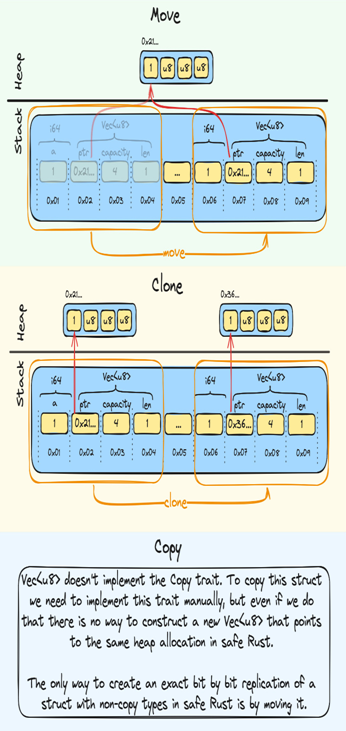
Figure 9.2 – Move, clone, and copy
A move will in many ways be like a deep copy of everything in our struct that’s located on the stack. This is problematic when you have a pointer that points to self, like we have with self-referential structs, since self will start at a new memory address after the move but the pointer to self won’t be adjusted to reflect that change.
Most of the time, when programming Rust, you probably won’t think a lot about moves since it’s part of the language you never explicitly use, but it’s important to know what it is and what it does.
Now that we’ve got a good understanding of what the problem is, let’s take a closer look at how Rust solves this by using its type system to prevent us from moving structs that rely on a stable place in memory to function correctly.